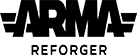 |
Arma Reforger Explorer
1.1.0.42
Arma Reforger Code Explorer by Zeroy - Thanks to MisterOutofTime
|
Go to the documentation of this file.
20 class BaseTimeAndWeatherManagerEntity: BaseWeatherManagerEntity
27 proto external
bool IsDayHour(
float hour24);
47 proto
float GetMoonPhaseForDate(
int year,
int month,
int day,
float timeOfTheDay24,
float timezone,
float dstOffset);
60 proto external
bool SetDate(
int year,
int month,
int day,
bool immediateChange =
false);
83 proto external
bool SetTimeOfTheDay(
float hours24,
bool immediateChange =
false);
130 proto external
int GetDay();
195 proto
bool GetSunriseHourForDate(
int year,
int month,
int day,
float latitude,
float longitude,
float timezone,
float dstOffset, out
float hour24);
200 proto
bool GetSunsetHourForDate(
int year,
int month,
int day,
float latitude,
float longitude,
float timezone,
float dstOffset, out
float hour24);
279 proto external
bool SetHoursMinutesSeconds(
int hours,
int minutes,
int seconds,
bool immediateChange =
false);
284 proto
void GetDate(out
int year, out
int month, out
int day);
307 static proto
void TimeToHoursMinutesSeconds(
float hours24, out
int hours, out
int minutes, out
int seconds);
321 static proto
float HoursMinutesSecondsToTime(
int hours,
int minutes,
int seconds);
384 proto external
void AddLightning(WeatherLightning lightning);
proto void SetTimeEvent(string time, Managed inst, func callback, bool delayed=true, bool singleUse=false)
proto external bool SetDSTEnabled(bool enabled)
proto external int GetWeekDayForDate(int year, int month, int day)
proto external int GetYear()
proto void GetCurrentSunMoonDirAndPhase(out vector outSunDir, out vector outMoonDir, out float outMoonPhase01)
proto external void GetWeatherStatesList(out notnull array< ref WeatherState > outStates)
proto bool GetSunsetHourForDate(int year, int month, int day, float latitude, float longitude, float timezone, float dstOffset, out float hour24)
proto external float GetWindSpeed()
proto external bool IsRainIntensityOverridden()
proto external bool SetHoursMinutesSeconds(int hours, int minutes, int seconds, bool immediateChange=false)
proto external bool IsNightHour(float hour24)
proto external int GetMonth()
proto external bool SetIsDayAutoAdvanced(bool autoAdvanced)
proto external float GetCurrentLatitude()
proto external float GetRainIntensity()
proto bool GetSunriseHourForDate(int year, int month, int day, float latitude, float longitude, float timezone, float dstOffset, out float hour24)
proto external bool IsWindSpeedOverridden()
proto float GetMoonPhaseForDate(int year, int month, int day, float timeOfTheDay24, float timezone, float dstOffset)
proto external bool IsWindDirectionOverridden()
proto external float GetMoonPhase(float timeOfTheDay24)
proto external bool SetDSTOffset(float dstOffsetHours)
proto external void UpdateWeather(float timeSlice)
proto external float GetTimeOfTheDay()
proto bool GetSunriseHour(out float hour24)
proto external bool CheckValidDate(int year, int month, int day)
proto external bool TryGetCompleteLocalWeather(LocalWeatherSituation lws, float swayFrequency, vector location)
proto void GetDate(out int year, out int month, out int day)
proto external float GetCurrentWetness()
proto external bool IsDayHour(float hour24)
proto external bool SetCurrentWetness(float wetness)
proto external float GetWindDirection()
proto external float GetCurrentWaterAccumulationPuddles()
proto external bool SetTimeOfTheDay(float hours24, bool immediateChange=false)
proto external bool SetDayDuration(float realtimeSeconds)
proto external bool SetCurrentLongitude(float longitude)
proto external bool SetDate(int year, int month, int day, bool immediateChange=false)
proto external bool GetIsDayAutoAdvanced()
proto external bool IsDSTEnabled()
proto external float GetCurrentWaterAccumulationCracks()
proto external float GetEngineTime()
proto external float GetTimeZoneOffset()
proto external float GetDSTOffset()
proto external void SetTimeZoneOffset(float utcTimeZone)
proto external float GetCurrentLongitude()
proto external bool SetWindSpeedOverride(bool doOverride, float windSpeed=0)
proto bool GetSunsetHour(out float hour24)
proto external float GetDayDuration()
proto external bool SetCurrentWaterAccumulation(float waterAccumulationCracks, float waterAccumulationPuddles)
proto external bool SetWindDirectionOverride(bool doOverride, float windDirection=0)
proto ref WeatherStateTransitionManager GetTransitionManager()
proto external bool SetCurrentLatitude(float latitude)
DownloadConfigCallback callback
BaseTimeAndWeatherManagerEntityClass BaseWeatherManagerEntityClass GetCurrentWeatherState()
proto external void AddLightning(WeatherLightning lightning)
proto external bool SetRainIntensityOverride(bool doOverride, float rainIntensity=0)
proto external int GetDay()
proto external int GetWeekDay()
proto void GetHoursMinutesSeconds(out int hours, out int minutes, out int seconds)