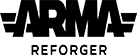 |
Arma Reforger Explorer
1.1.0.42
Arma Reforger Code Explorer by Zeroy - Thanks to MisterOutofTime
|
Go to the documentation of this file.
36 [
RplProp(onRplName:
"OnFlashesRpl")]
39 [
RplProp(onRplName:
"OnFlashesRpl")]
42 [
RplProp(onRplName:
"OnFlashesRpl")]
45 [
RplProp(onRplName:
"OnFlashesRpl")]
48 [
RplProp(onRplName:
"OnFlashesRpl")]
63 TimeAndWeatherManagerEntity tawme =world.GetTimeAndWeatherManager();
68 WeatherLightning wl =
new WeatherLightning();
74 WeatherLightningFlash wlf =
new WeatherLightningFlash();
80 wl.AddLightningFlash(wlf);
83 tawme.AddLightning(wl);
94 RplComponent rpl = RplComponent.Cast(owner.FindComponent(RplComponent));
100 TimeAndWeatherManagerEntity tawme = world.GetTimeAndWeatherManager();
104 if(rpl && rpl.Role() == RplRole.Authority)
112 float curEngineTime = tawme.GetEngineTime();
116 float flashStartTime = curEngineTime;
120 curEngineTime += (flashDuration + flashCooldownDuration);
protected float m_fFlashMaxDurationMillis
SCR_FragmentEntityClass ComponentEditorProps
protected int m_iMinFlashes
SCR_SiteSlotEntityClass ScriptComponent
protected ref array< float > m_aFlashesStartTime
SCR_RplTestEntityClass RplProp
Used for handling entity spawning requests for SCR_CatalogEntitySpawnerComponent and inherited classe...
protected float m_fFlashMinDurationMillis
protected float m_fFlashMinVisibilityRadiusKM
SCR_LightningComponentClass m_pRandomGenerator
Inform the weather manager a lightning has been spawned. Weather Manager will handle light changes.
protected int m_iMaxFlashes
typedef Attribute
Post-process effect of scripted camera.
protected int m_iNumFlashes
override void OnPostInit(IEntity owner)
Called on PostInit when all components are added.
protected float m_fFlashMaxCooldownMillis
IEntity GetOwner()
Owner entity of the fuel tank.
protected ref array< float > m_aFlashesDuration
protected float m_fFlashMaxVisibilityRadiusKM
protected ref array< float > m_aFlashesCooldownDuration
protected float m_fFlashMinCooldownMillis
protected int m_iCurFlashIndex
protected float m_fRadiusKM
SCR_PossessingManagerComponentClass int