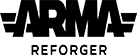 |
Arma Reforger Explorer
1.1.0.42
Arma Reforger Code Explorer by Zeroy - Thanks to MisterOutofTime
|
ScriptInvokerBase< ScriptInvokerString5Method > ScriptInvokerString5
ScriptInvokerBase< ScriptInvokerEntity2Method > ScriptInvokerEntity2
func ScriptInvokerString2Method
ScriptInvokerBase< ScriptInvokerFloat3Method > ScriptInvokerFloat3
func ScriptInvokerString4Method
func ScriptInvokerFloat5Method
ScriptInvokerBase< ScriptInvokerInt3Method > ScriptInvokerInt3
ScriptInvokerBase< ScriptInvokerInt2Method > ScriptInvokerInt2
ScriptInvokerBase< ScriptInvokerString4Method > ScriptInvokerString4
ScriptInvokerBase< ScriptInvokerInt4Method > ScriptInvokerInt4
ScriptInvokerBase< ScriptInvokerIntMethod > ScriptInvokerInt
ScriptInvokerBase< ScriptInvokerBool2Method > ScriptInvokerBool2
func ScriptInvokerSCRScriptedWidgetComponentMethod
func ScriptInvokerEntityAndStorageMethod
func ScriptInvokerStringMethod
ScriptInvokerBase< ScriptInvokerFloat2Method > ScriptInvokerFloat2
ScriptInvokerBase< ScriptInvokerString3Method > ScriptInvokerString3
ScriptInvokerBase< ScriptInvokerBool3Method > ScriptInvokerBool3
func ScriptInvokerString3Method
func ScriptInvokerFloat4Method
func ScriptInvokerBool2Method
func ScriptInvokerWidgetMethod
func ScriptInvokerInt4Method
func ScriptInvokerBaseWorldMethod
func ScriptInvokerIntMethod
func ScriptInvokerInt5Method
ScriptInvokerBase< ScriptInvokerFloatMethod > ScriptInvokerFloat
func ScriptInvokerVectorMethod
ScriptInvokerBase< ScriptInvokerBoolMethod > ScriptInvokerBool
ScriptInvokerBase< ScriptInvokerStringMethod > ScriptInvokerString
ScriptInvokerBase< ScriptInvokerBaseWorldMethod > ScriptInvokerBaseWorld
func ScriptInvokerEntity2Method
ScriptInvokerBase< ScriptInvokerEntityMethod > ScriptInvokerEntity
func ScriptInvokerRplIdMethod
func ScriptInvokerString5Method
func ScriptInvokerBool4Method
ScriptInvokerBase< ScriptInvokerBool4Method > ScriptInvokerBool4
func ScriptInvokerInt2Method
ScriptInvokerBase< ScriptInvokerEntityAndStorageMethod > ScriptInvokerEntityAndStorage
ScriptInvokerBase< ScriptInvokerString2Method > ScriptInvokerString2
ScriptInvokerBase< ScriptInvokerSCRScriptedWidgetComponentMethod > ScriptInvokerSCRScriptedWidgetComponent
ScriptInvokerBase< ScriptInvokerVectorMethod > ScriptInvokerVector
func ScriptInvokerFloatMethod
ScriptInvokerBase< ScriptInvokerFloat4Method > ScriptInvokerFloat4
func ScriptInvokerFloat3Method
func ScriptInvokerFloat2Method
ScriptInvokerBase< ScriptInvokerVoidMethod > ScriptInvokerVoid
func ScriptInvokerEntityMethod
ScriptInvokerBase< ScriptInvokerWidgetMethod > ScriptInvokerWidget
func ScriptInvokerBool3Method
ScriptInvokerBase< ScriptInvokerInt5Method > ScriptInvokerInt5
ScriptInvokerBase< ScriptInvokerFloat5Method > ScriptInvokerFloat5
func ScriptInvokerInt3Method
ScriptInvokerBase< ScriptInvokerRplIdMethod > ScriptInvokerRplId
func ScriptInvokerBool5Method
func ScriptInvokerBoolMethod
ScriptInvokerBase< ScriptInvokerBool5Method > ScriptInvokerBool5
func ScriptInvokerVoidMethod