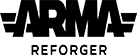 |
Arma Reforger Explorer
1.1.0.42
Arma Reforger Code Explorer by Zeroy - Thanks to MisterOutofTime
|
Go to the documentation of this file.
16 class VehicleWheeledSimulation_SA: VehicleBaseSimulation
41 proto external
void SetBreak(
float in,
bool hb);
50 proto external
void SetGear(
int in);
109 proto external vector
WheelGetPosition(
int wheelIdx,
float displacement = 0.0);
proto external EWheelContactLiquidState WheelGetContactLiquidState(int wheelIdx)
Returns whether and how is wheel in contact with some liquid.
proto external float GetNoiseSteerSensitivity()
Returns sensitivity against noise steer value of surface in range < 0, 1 >
proto external float EngineGetRPM()
Returns current engine's RPM.
proto external void EngineStop()
Stops the engine.
proto external void WheelTyreSetRoughnessState(int wheelIdx, float roughness)
Sets current roughness of wheel's tyre surface.
proto external bool WheelHasContact(int wheelIdx)
Returns true if wheel has contact with ground or other object.
proto external int WheelGetIndex(string name)
Returns stored index of the wheel based on its name.
VehicleWheeledSimulationClass VehicleBaseSimulationClass GetSpeedKmh()
Returns current vehicle speed in km/h (kilometres per hour).
proto external int GetGear()
Returns index of currently engaged gear.
proto external float GetThrottle()
returns current throttle input in range < 0, 1 >
proto external float EngineGetRPMFeedback()
Returns RPM that is feeded back from rest of the powertrain.
proto external int GearboxForwardGearsCount()
Returns number of forward gears.
proto external vector WheelGetContactNormal(int wheelIdx)
Returns wheel contact normal.
proto external float EngineGetRPMPeakTorque()
Returns rpm at which engine provides maximal torque.
proto external float WheelTyreGetRoughnessState(int wheelIdx)
Returns current roughness of wheel's tyre surface.
proto external float WheelGetRPM(int wheelIdx)
Returns current wheel's RPM.
proto external bool IsHandbrakeOn()
Returns whether handbrake is currently applied.
proto external void SetBreak(float in, bool hb)
proto external int GearboxGearsCount()
Returns number of all gear ratios (neutral included).
proto external void EngineSetPeakTorqueState(float peakTorque)
proto external GameMaterial WheelGetContactMaterial(int wheelIdx)
Returns wheel contact material.
proto external float EngineGetPeakTorqueState()
Returns current engine's peak torque in Nm (newton-metre).
proto external void SetSteering(float in)
proto external float WheelTyreGetRoughness(int wheelIdx)
Returns initial roughness of wheel's tyre surface.
proto external void EngineSetPeakPowerState(float peakPower)
proto external float GetSteering()
Returns current steering input in range < -1, 1 >
proto external void WheelSetRollingDrag(int wheelIdx, float drag)
Sets current rolling drag percentage.
proto external float WheelSetRadiusState(int wheelIdx, float radius)
Sets current wheel's radius.
proto external float WheelTyreGetLateralFrictionState(int wheelIdx)
Returns tyre's current lateral friction.
proto external void SetNoiseSteerSensitivity(float newValue)
proto external GameMaterial WheelGetContactLiquidMaterial(int wheelIdx)
Returns the material of the liquid which the wheel is in contact.
proto external void WheelTyreSetLateralFrictionState(int wheelIdx, float latFriction)
Sets tyre's current lateral friction.
proto external vector WheelGetContactPosition(int wheelIdx)
Returns wheel contact position in world space.
proto external float WheelGetRadiusState(int wheelIdx)
Returns current wheel's radius.
proto external float GetClutch()
proto external void GearboxSetEfficiencyState(float efficiency)
Sets current gearbox efficiency.
proto external float GearboxGetEfficiency()
Returns initial gearbox efficiency.
proto external float EngineGetRPMIdle()
Returns idle rpm of the engine.
proto external void WheelTyreSetLongitudinalFrictionState(int wheelIdx, float lngFriction)
Sets tyre's current longitudinal friction.
proto external float WheelGetMass(int wheelIdx)
Returns initial wheel's mass.
proto external float GearboxGetEfficiencyState()
Returns current gearbox efficiency.
proto external vector WheelGetPosition(int wheelIdx, float displacement=0.0)
proto external float WheelGetLongitudinalSlip(int wheelIdx)
Returns longitudinal slip of tyre.
proto external void ForceEnableSimulation()
proto external IEntity WheelGetContactEntity(int wheelIdx)
Returns entity which is in contact with wheel.
proto external float GetBrake()
Returns current brake input in range < 0, 1 >
proto external float EngineGetPeakTorque()
Returns initial engine's peak torque in Nm (newton-metre).
proto external float WheelGetRollingDrag(int wheelIdx)
Returns current wheel's rolling drag percentage.
proto external float WheelGetLateralSlip(int wheelIdx)
Returns lateral slip of tyre.
proto external float WheelGetRadius(int wheelIdx)
Returns initial wheel's radius.
EWheelContactLiquidState
Describes whether wheel is in contact with some liquid.
proto external string WheelGetName(int wheelIdx)
Returns name of the wheel based on its index.
proto external void SetClutch(float in)
proto external bool EngineIsOn()
Returns true if engine is running.
VehicleHelicopterSimulationClass VehicleBaseSimulationClass SetThrottle(float in)
proto external float GetRoughnessSensitivity()
Returns sensitivity against roughness value of surface in range < 0, 1 >
proto external float WheelGetMassState(int wheelIdx)
Returns current wheel's mass.
proto external void SetRoughnessSensitivity(float newValue)
proto external int WheelCount()
Returns number of wheels.
proto external float WheelTyreGetLongitudinalFriction(int wheelIdx)
Returns tyre's initial longitudinal friction.
proto external float EngineGetRPMMax()
Returns maximal working rpm before engine explodes.
proto external void EngineStart()
Starts the engine.
proto external float EngineGetPeakPowerState()
Returns current engine's peak power in kW (kilowatts).
proto external void SetGear(int in)
Switches to given gear.
proto external float EngineGetRPMPeakPower()
Returns rpm at which engine provides maximal power.
proto external float EngineGetPeakPower()
Returns initial engine's peak power in kW (kilowatts).
proto external vector WheelGetContactLiquidPosition(int wheelIdx)
Returns the position where the wheel touches a liquid surface.
proto external float WheelTyreGetLongitudinalFrictionState(int wheelIdx)
Returns tyre's current longitudinal friction.
proto external float EngineGetLoad()
Returns current engine load in range < 0, 1 >
proto external float WheelTyreGetLateralFriction(int wheelIdx)
Returns tyre's initial lateral friction.
proto external float WheelSetMassState(int wheelIdx, float mass)
Sets current wheel's mass.