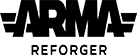 |
Arma Reforger Explorer
1.1.0.42
Arma Reforger Code Explorer by Zeroy - Thanks to MisterOutofTime
|
Go to the documentation of this file.
12 protected int m_iItemOwnerID = -1;
19 void Deploy(IEntity userEntity =
null)
29 m_iItemOwnerID = playerManager.GetPlayerIdFromControlledEntity(userEntity);
87 return m_iItemOwnerID;
93 super.OnPostInit(owner);
94 SetEventMask(
GetOwner(), EntityEvent.INIT);
enum EQueryType EntityEditorProps(category:"GameScripted/Sound", description:"THIS IS THE SCRIPT DESCRIPTION.", color:"0 0 255 255")
protected bool m_bIsDeployed
SCR_SiteSlotEntityClass ScriptComponent
bool CanDismantleBeShown(notnull IEntity userEntity)
ArmaReforgerScripted GetGame()
void Dismantle(IEntity userEntity=null)
SCR_BaseDeployableInventoryItemComponentClass ScriptComponentClass RplProp(onRplName:"OnRplDeployed")
Base class which all deployable inventory items inherit from.
override void OnPostInit(IEntity owner)
Called on PostInit when all components are added.
IEntity GetOwner()
Owner entity of the fuel tank.
protected void OnRplDeployed()
protected RplComponent m_RplComponent
bool CanDeployBeShown(notnull IEntity userEntity)
bool Deploy(SCR_EMobileAssemblyStatus status, int playerId=0)