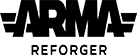 |
Arma Reforger Explorer
1.1.0.42
Arma Reforger Code Explorer by Zeroy - Thanks to MisterOutofTime
|
Go to the documentation of this file. 1 [
ComponentEditorProps(
category:
"GameScripted/Editor (Editables)", description:
"", icon:
"WBData/ComponentEditorProps/componentEditor.png")]
11 [
RplProp(onRplName:
"OnPreWaypointIdRpl")]
12 protected RplId m_PrevWaypointId;
15 protected bool m_bIsCurrent;
31 m_bIsCurrent = isCurrent;
32 m_PrevWaypoint = prevWaypoint;
33 m_PrevWaypointId = Replication.FindId(prevWaypoint);
59 return m_PrevWaypoint;
71 override bool CanDuplicate(out notnull set<SCR_EditableEntityComponent> outRecipients)
75 outRecipients.Insert(groupComponent);
112 super.OnParentEntityChanged(group, parentEntityPrev, changedByUser);
122 return super.GetPos(pos);
132 AIGroup group = AIGroup.Cast(recipient.GetOwner());
139 editableGroup.ClearWaypoints();
142 group.AddWaypoint(AIWaypoint.Cast(
GetOwner()));
151 super.OnDelete(owner);
156 AIGroup aiGroup = AIGroup.Cast(
m_Group.GetOwner());
158 aiGroup.RemoveWaypoint(AIWaypoint.Cast(owner));
SCR_FragmentEntityClass ComponentEditorProps
override void OnDelete(IEntity owner)
protected SCR_AIGroup m_Group
void SCR_EditableGroupComponent(IEntityComponentSource src, IEntity ent, IEntity parent)
override bool CanDuplicate(out notnull set< SCR_EditableEntityComponent > outRecipients)
override bool GetPos(out vector pos)
override Faction GetFaction()
SCR_EditableWaypointComponentClass SCR_EditableEntityComponentClass RplProp(onRplName:"OnPreWaypointIdRpl")
Special configuration for editable waypoint.
protected void OnPreWaypointIdRpl()
EEditableEntityType
Defines type of SCR_EditableEntityComponent. Assigned automatically based on IEntity inheritance.
override SCR_EditableEntityComponent EOnEditorPlace(out SCR_EditableEntityComponent parent, SCR_EditableEntityComponent recipient, EEditorPlacingFlags flags, bool isQueue, int playerID=0)
IEntity GetOwner()
Owner entity of the fuel tank.
SCR_DestructionSynchronizationComponentClass ScriptComponentClass int index
SCR_EditableEntityComponent GetPrevWaypoint()
override void OnParentEntityChanged(SCR_EditableEntityComponent parentEntity, SCR_EditableEntityComponent parentEntityPrev, bool changedByUser)
override SCR_EditableEntityComponent GetAIGroup()