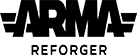 |
Arma Reforger Explorer
1.1.0.42
Arma Reforger Code Explorer by Zeroy - Thanks to MisterOutofTime
|
Go to the documentation of this file. 1 [
EntityEditorProps(
category:
"GameScripted/Campaign", description:
"This allows only vehicle requester enther the vehicle as driver for defined period of time.")]
6 class SCR_VehicleSpawnProtectionComponent : SCR_BaseLockComponent
8 protected static const int NO_OWNER = -1;
9 protected static const int NO_TIME_LIMIT = 0;
18 [
Attribute(defvalue:
"#AR-Campaign_Action_CannotEnterVehicle-UC",
desc:
"Text that will be displayed on actions telling reason why it cannot be used")];
24 SetEventMask(owner, EntityEvent.INIT);
30 ClearEventMask(owner, EntityEvent.INIT);
48 if (protectionTime != NO_TIME_LIMIT)
67 IEntity playerEnt =
GetGame().GetPlayerManager().GetPlayerControlledEntity(playerID);
113 bool IsProtected(notnull IEntity playerEntering, notnull BaseCompartmentSlot compartmentSlot)
119 int testPlayerID = SCR_PossessingManagerComponent.GetPlayerIdFromMainEntity(playerEntering);
124 Print(
string.Format(
"Player ID is %1, vehicle owner ID is %2. Vehicle is unlocked.", testPlayerID,
m_iVehicleOwnerID), LogLevel.NORMAL);
129 Print(
string.Format(
"Player ID is %1, vehicle owner ID is %2. Vehicle is LOCKED.", testPlayerID,
m_iVehicleOwnerID), LogLevel.NORMAL);
135 [
Obsolete(
"Use OnPlayerDisconnected instead")]
SCR_VehicleSpawnProtectionComponentClass NO_OWNER
enum EQueryType EntityEditorProps(category:"GameScripted/Sound", description:"THIS IS THE SCRIPT DESCRIPTION.", color:"0 0 255 255")
void SetReasonText(string text)
protected void OnPlayerDisconnected(int playerID)
SCR_RplTestEntityClass RplProp
Used for handling entity spawning requests for SCR_CatalogEntitySpawnerComponent and inherited classe...
ArmaReforgerScripted GetGame()
UI Textures DeployMenu Briefing conflict_HintBanner_1_UI desc
SCR_BaseGameMode GetGameMode()
protected void OnPlayerDisconected(int playerID)
protected int m_iTimeOfProtection
typedef Attribute
Post-process effect of scripted camera.
override void OnPostInit(IEntity owner)
Called on PostInit when all components are added.
LocalizedString GetReasonText(IEntity user)
void SetProtectionTime(int protectionTime)
override void EOnInit(IEntity owner)
void SetProtectOnlyDriverSeat(bool onlyDriverSeat)
void RemoveEventHandlers()
private int m_iVehicleOwnerID
bool IsProtected(notnull IEntity playerEntering, notnull BaseCompartmentSlot compartmentSlot)
void SetVehicleOwner(int playerID)
private SCR_CharacterControllerComponent m_CharControlComp
RespawnSystemComponentClass GameComponentClass Obsolete()] proto external GenericEntity DoSpawn(string prefab
RespawnSystemComponent should be attached to a gamemode to handle player spawning and respawning.
protected string m_sReasonText