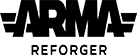 |
Arma Reforger Explorer
1.1.0.42
Arma Reforger Code Explorer by Zeroy - Thanks to MisterOutofTime
|
Go to the documentation of this file. 1 [
EntityEditorProps(
category:
"GameScripted/Campaign", description:
"Makes a vehicle able to carry Conflict resources.", color:
"0 0 255 255")]
16 protected static const float SUPPLY_TRUCK_UNLOAD_RADIUS = 25;
29 [
Attribute(
"0",
desc:
"How many supplies this component holds when it is created."),
RplProp(onRplName:
"OnSuppliesChanged")]
33 [
Attribute(
"0",
desc:
"Maximum supplies this component can hold."),
RplProp(onRplName:
"OnSuppliesChanged")]
36 [
Attribute(
"0",
desc:
"Maximum distance from a supply depot a player can still (un)load their truck")]
39 [
Attribute(
"0", UIWidgets.CheckBox,
"This component belongs to a supply depot without a parent base.",
"")]
45 [
Obsolete(
"Use SCR_ResourceComponent operations instead")]
48 array<int> loadingArray;
56 if(!loadingArray.IsEmpty())
57 return loadingArray[0];
64 [
Obsolete(
"Use SCR_ResourceComponent operations instead")]
78 [
Obsolete(
"Use SCR_ResourceComponent operations instead")]
99 [
Obsolete(
"Use SCR_ResourceComponent operations instead")]
105 Replication.BumpMe();
112 [
Obsolete(
"Use SCR_ResourceComponent operations instead")]
118 Replication.BumpMe();
123 [
Obsolete(
"Use SCR_ResourceComponent operations instead")]
132 [
Obsolete(
"Use SCR_ResourceComponent operations instead")]
140 [
Obsolete(
"Use SCR_ResourceComponent operations instead")]
148 [
Obsolete(
"Use SCR_ResourceComponent operations instead")]
155 Replication.BumpMe();
162 [
Obsolete(
"Use SCR_ResourceComponent operations instead")]
168 return SUPPLY_TRUCK_UNLOAD_RADIUS;
173 [
Obsolete(
"Use SCR_ResourceComponent operations instead")]
181 [
Obsolete(
"Use SCR_ResourceComponent operations instead")]
189 [
Obsolete(
"Use SCR_ResourceComponent operations instead")]
202 [
Obsolete(
"Use SCR_ResourceComponent operations instead")]
216 [
Obsolete(
"Use SCR_ResourceComponent operations instead")]
226 [
Obsolete(
"Use SCR_ResourceComponent operations instead")]
239 Replication.BumpMe();
246 [
Obsolete(
"Use SCR_ResourceComponent operations instead")]
268 campaign.GetBaseManager().RegisterRemnantSupplyDepot(
this);
275 super.OnPostInit(owner);
276 SetEventMask(owner, EntityEvent.INIT);
280 string err =
string.Format(
"SCR_CampaignSuppliesComponent on %1 carries more supplies (%2) than its maximum (%3)!", owner,
m_iSupplies,
m_iSuppliesMax);
281 Print(err, LogLevel.ERROR);
293 if (suppliesComponent)
294 return suppliesComponent;
296 SlotManagerComponent slotManager = SlotManagerComponent.Cast(ent.FindComponent(SlotManagerComponent));
300 array<EntitySlotInfo> slots = {};
301 slotManager.GetSlotInfos(slots);
309 truckBed = slot.GetAttachedEntity();
315 if (suppliesComponent)
316 return suppliesComponent;
enum EQueryType EntityEditorProps(category:"GameScripted/Sound", description:"THIS IS THE SCRIPT DESCRIPTION.", color:"0 0 255 255")
SCR_SiteSlotEntityClass ScriptComponent
void SetSupplyLoadingPlayer(int playerID)
protected bool m_bIsPlayerInRange
SCR_RplTestEntityClass RplProp
Used for handling entity spawning requests for SCR_CatalogEntitySpawnerComponent and inherited classe...
ArmaReforgerScripted GetGame()
protected int m_iSuppliesMax
protected ref array< int > m_aLoadingPlayerIDs
void DeleteSupplyLoadingPlayer(int playerID)
UI Textures DeployMenu Briefing conflict_HintBanner_1_UI desc
protected ref array< int > m_aUnloadingPlayerIDs
SCR_CampaignSuppliesComponentClass m_LastLoadedAt
Makes a vehicle able to carry Conflict resources.
void SetLastUnloadedAt(SCR_CampaignMilitaryBaseComponent base)
Adds ability to attach an object to a slot.
protected SCR_CampaignMilitaryBaseComponent m_LastXPAwardedAt
bool GetIsStandaloneDepot()
SCR_BaseGameMode GetGameMode()
void SCR_CampaignSuppliesComponent(IEntityComponentSource src, IEntity ent, IEntity parent)
void SCR_GameModeCampaign(IEntitySource src, IEntity parent)
bool m_bIsStandaloneDepot
void DeleteSupplyUnloadingPlayer(int playerID)
typedef Attribute
Post-process effect of scripted camera.
protected float m_fOperationalRadius
protected void OnPlayerKilled(int playerId, IEntity playerEntity, IEntity killerEntity, notnull Instigator killer)
protected bool m_bAwardUnloadXP
override void OnPostInit(IEntity owner)
Called on PostInit when all components are added.
float GetOperationalRadius()
void SetLastLoadedAt(SCR_CampaignMilitaryBaseComponent base)
IEntity GetOwner()
Owner entity of the fuel tank.
void SetSuppliesMax(int suppliesMax)
void SetIsPlayerInRange(bool status)
SCR_CampaignSuppliesComponent GetSuppliesComponent()
override void EOnInit(IEntity owner)
ref ScriptInvoker m_OnSuppliesChanged
bool GetIsPlayerInRange()
protected int m_iSupplies
void ~SCR_CampaignSuppliesComponent()
void SetSupplyUnloadingPlayer(int playerID)
ref ScriptInvoker m_OnSuppliesTruckDeleted
int GetLoadingPlayer(bool unloading=false)
protected SCR_CampaignMilitaryBaseComponent m_LastUnloadedAt
RespawnSystemComponentClass GameComponentClass Obsolete()] proto external GenericEntity DoSpawn(string prefab
RespawnSystemComponent should be attached to a gamemode to handle player spawning and respawning.
void AddSupplies(int supplies, bool replicate=true)