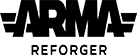 |
Arma Reforger Explorer
1.1.0.42
Arma Reforger Code Explorer by Zeroy - Thanks to MisterOutofTime
|
Go to the documentation of this file.
47 super.UpdateSoundJob(owner, timeSlice);
66 Physics physics = owner.GetPhysics();
70 vector cameraTransform[4];
71 GetGame().GetWorld().GetCurrentCamera(cameraTransform);
73 vector entityPos = owner.GetOrigin();
74 vector entityVelocity = physics.GetVelocity();
76 vector directionCameraEntity = (cameraTransform[3] - entityPos).Normalized();
77 float speed = vector.Dot(entityVelocity, directionCameraEntity);
102 owner.GetTransform(mat);
103 mat[3][1] = mat[3][1] - altitudeAGL;
137 SCR_DamageManagerComponent hitZoneContainerComponent = SCR_DamageManagerComponent.Cast(
GetOwner().FindComponent(SCR_DamageManagerComponent));
138 if (!hitZoneContainerComponent)
150 SCR_DamageManagerComponent hitZoneContainerComponent = SCR_DamageManagerComponent.Cast(
GetOwner().FindComponent(SCR_DamageManagerComponent));
151 if (!hitZoneContainerComponent)
174 super.OnPostInit(owner);
const protected string ROTOR_MAIN_DAMAGE_STATE_SIGNAL_NAME
enum EQueryType EntityEditorProps(category:"GameScripted/Sound", description:"THIS IS THE SCRIPT DESCRIPTION.", color:"0 0 255 255")
void SCR_VehicleSoundComponent(IEntityComponentSource src, IEntity ent, IEntity parent)
protected void HandleWashRotor(IEntity owner)
protected AudioHandle m_WashRotorAudioHandle
Wash rotor audio handle.
protected int m_iDistanceSignalIdx
protected int m_AltitudeAGLSignalIdx
ArmaReforgerScripted GetGame()
void ~SCR_HelicopterSoundComponent()
protected void RegisterOnDamageChanged()
protected void OnDamageStateChanged(EDamageState state)
protected int m_MainRotorRPMScaledIdx
protected SCR_HelicopterDamageManagerComponent m_HelicopterDamageManagerComponent
Damage state signal.
const protected string DAMAGE_STATE_SIGNAL_NAME
SoundComponentClass SimpleSoundComponentClass SoundEvent(string eventName)
Play a sound from the owner entity's position.
const protected string ALTITUDE_AGL
protected void UnregisterRotorMainOnDamageChanged()
const protected float ALTITUDE_LIMIT
protected EDamageState m_eRotorMainDamageState
override void OnPostInit(IEntity owner)
Called on PostInit when all components are added.
const protected float MAIN_ROTOR_RPM_SCALED_THRESHOLD
protected void RotorMainOnStateChanged()
protected int m_iSpeedToCameraSignalIdx
protected SCR_HitZone m_RotorMainHitZone
Main rotor damage state.
IEntity GetOwner()
Owner entity of the fuel tank.
protected SignalsManagerComponent m_SignalsManagerComponent
const protected float WASH_ROTOR_DISTANCE_LIMIT
const protected float UPDATE_TIME
const protected string DISTANCE_SIGNAL_NAME
SCR_HelicopterSoundComponentClass SPEED_TO_CAMERA_SIGNAL_NAME
protected void UnregisterOnDamageChanged()
override void UpdateSoundJob(IEntity owner, float timeSlice)
Call when component is in range.
protected void RegisterRotorMainOnDamageChanged()
override void OnInit(IEntity owner)
protected void CalculateSpeedToCameraSignal(IEntity owner)
const protected string MAIN_ROTOR_RPM_SCALED
const protected string MAIN_ROTOR_HIT_ZONE_NAME