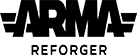 |
Arma Reforger Explorer
1.1.0.42
Arma Reforger Code Explorer by Zeroy - Thanks to MisterOutofTime
|
Go to the documentation of this file.
16 class SCR_InfoDisplayExtended : SCR_InfoDisplay
18 [
Attribute(
"1", UIWidgets.CheckBox,
"Toggles ON/OFF info display.")]
21 [
Attribute(
"39", UIWidgets.Flags,
"Defines when the GUI element is allowed to show.",
"", ParamEnumArray.FromEnum(
EShowGUI) )]
44 #ifdef DEBUG_INFO_DISPLAY_EXT
46 void _printClass(
string str)
51 Print(str, LogLevel.DEBUG);
91 #ifdef DEBUG_INFO_DISPLAY_EXT
92 _printClass(
string.Format(
"%1 [DisplayOnSuspended]",
this));
101 #ifdef DEBUG_INFO_DISPLAY_EXT
102 _printClass(
string.Format(
"%1 [DisplayOnResumed]",
this));
112 #ifdef DEBUG_INFO_DISPLAY_EXT
113 _printClass(
string.Format(
"%1 [OnStartDraw] owner: %2",
this, owner));
163 core.Event_OnEditorManagerInitOwner.Insert(
OnEditorInit);
191 super.OnStartDraw(owner);
215 #ifdef DEBUG_INFO_DISPLAY_EXT
216 _printClass(
string.Format(
"%1 [OnControlledEntityChanged] %2 -> %3",
this, from, to));
221 ChimeraCharacter character = ChimeraCharacter.Cast(from);
231 m_CameraHandler = SCR_CharacterCameraHandlerComponent.Cast(to.FindComponent(SCR_CharacterCameraHandlerComponent));
241 IEntity eventHandlerOwner;
243 eventHandlerOwner = to;
247 if (eventHandlerOwner)
248 m_EventHandlerManager = EventHandlerManagerComponent.Cast(eventHandlerOwner.FindComponent(EventHandlerManagerComponent));
258 ChimeraCharacter character = ChimeraCharacter.Cast(to);
290 #ifdef DEBUG_INFO_DISPLAY_EXT
291 _printClass(
string.Format(
"%1 [OnADSSwitched] inADS: %2",
this, inADS));
302 #ifdef DEBUG_INFO_DISPLAY_EXT
303 _printClass(
string.Format(
"%1 [OnLifeStateChanged] lifeState: %2",
this,
SCR_Enum.GetEnumName(
ECharacterLifeState, newLifeState)));
316 #ifdef DEBUG_INFO_DISPLAY_EXT
317 _printClass(
string.Format(
"%1 [OnPauseMenuOpen]",
this));
328 #ifdef DEBUG_INFO_DISPLAY_EXT
329 _printClass(
string.Format(
"%1 [OnPauseMenuClose]",
this));
340 #ifdef DEBUG_INFO_DISPLAY_EXT
341 _printClass(
string.Format(
"%1 [OnEditorInit] editorManager: %2",
this, editorManager));
362 #ifdef DEBUG_INFO_DISPLAY_EXT
363 _printClass(
string.Format(
"%1 [OnEditorOpen]",
this));
374 #ifdef DEBUG_INFO_DISPLAY_EXT
375 _printClass(
string.Format(
"%1 [OnEditorClose]",
this));
386 #ifdef DEBUG_INFO_DISPLAY_EXT
406 bool updateNeeded = (cameraPassed && statePassed && menuPassed && editorPassed) !=
m_bCanShow;
408 m_bCanShow = cameraPassed && statePassed && menuPassed && editorPassed;
422 override void Show(
bool show,
float speed =
UIConstants.FADE_RATE_INSTANT, EAnimationCurve curve = EAnimationCurve.LINEAR)
428 #ifdef DEBUG_INFO_DISPLAY_EXT
429 _printClass(
string.Format(
"%1 [Show] m_bShown && m_bCanShow: %2 | m_bShown: %3 | m_bCanShow: %4",
this, show &&
m_bCanShow, show,
m_bCanShow));
440 #ifdef DEBUG_INFO_DISPLAY_EXT
441 _printClass(
string.Format(
"%1 [OnStopDraw] owner: %2",
this, owner));
472 super.OnStopDraw(owner);
478 private override event void OnInit(IEntity owner)
480 #ifdef DEBUG_INFO_DISPLAY_EXT
481 _printClass(
string.Format(
"%1 [OnInit] owner: %2",
this, owner));
499 private override event void UpdateValues(IEntity owner,
float timeSlice)
504 super.UpdateValues(owner, timeSlice);
private void OnEditorOpen()
private void OnADSSwitched(BaseWeaponComponent weapon, bool inADS)
private override event void OnStopDraw(IEntity owner)
protected SCR_EditorManagerEntity m_EditorManager
private void OnEditorInit(SCR_EditorManagerEntity editorManager)
private override event void OnStartDraw(IEntity owner)
protected void DisplayStartDraw(IEntity owner)
private void OnControlledEntityChanged(IEntity from, IEntity to)
Runs every time the controlled entity has been changed.
protected SCR_HUDManagerComponent m_HUDManager
ArmaReforgerScripted GetGame()
protected void DisplayUpdate(IEntity owner, float timeSlice)
protected bool m_bInThirdPerson
protected void DisplayOnResumed()
protected bool m_bShowInAllCameras
private override event void UpdateValues(IEntity owner, float timeSlice)
protected void DisplayStopDraw(IEntity owner)
protected EventHandlerManagerComponent m_EventHandlerManager
protected bool m_bCanShow
void SetEnabled(bool isEnabled)
override void Show(bool show, float speed=UIConstants.FADE_RATE_INSTANT, EAnimationCurve curve=EAnimationCurve.LINEAR)
protected void DisplayInit(IEntity owner)
Core component to manage SCR_EditorManagerEntity.
protected void DisplayControlledEntityChanged(IEntity from, IEntity to)
protected SCR_CharacterControllerComponent m_CharacterController
protected bool m_bIsEnabled
protected SCR_CharacterCameraHandlerComponent m_CameraHandler
private void UpdateVisibility()
enum EShowGUI Attribute("1", UIWidgets.CheckBox, "Toggles ON/OFF info display.")] protected bool m_bIsEnabled
private void OnPauseMenuOpen()
private void OnPauseMenuClose()
private void OnEditorClose()
protected bool m_bIsUnconscious
protected bool m_bAttachedToPlayerController
protected void DisplayConsciousnessChanged(bool conscious, bool init=false)
protected bool m_bInEditor
protected void DisplayOnSuspended()
Called when GUI is temporarily suspended due to visibility flags; e.g. GM entered and GUI marked as n...
protected bool m_bInPauseMenu
private override event void OnInit(IEntity owner)
protected SCR_PlayerController m_PlayerController
protected MenuManager m_MenuManager
private void OnLifeStateChanged(ECharacterLifeState previousLifeState, ECharacterLifeState newLifeState)
Will be called when the life state of the character changes.
protected bool DisplayStartDrawInit(IEntity owner)
SCR_AIUtilityComponentClass m_OwnerEntity