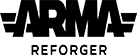 |
Arma Reforger Explorer
1.1.0.42
Arma Reforger Code Explorer by Zeroy - Thanks to MisterOutofTime
|
Go to the documentation of this file.
14 [
Attribute(
"", UIWidgets.Object,
desc:
"Array of x*y sizes in unscaled pix")]
15 protected ref array<ref ToolSize> m_aSizesArray;
17 protected static const string BASE_WORLD_TYPE =
"ChimeraWorld";
18 protected static const float CAMERA_VERTICAL_FOV = 43;
23 protected string WORLD_RESOURCE =
"{88ABCDC0EEC969DF}Prefabs/World/PreviewWorld/MapCompassWorld.et";
116 ScriptCallQueue queue =
GetGame().GetCallqueue();
153 World previewRTWorld =
m_RTWorld.GetRef();
154 previewRTWorld.ReloadSystems();
157 BaseWorld previewWorld =
m_RTWorld.GetRef();
166 GetGame().SpawnEntityPrefabLocal(rsc, previewWorld);
169 previewWorld.SetCameraType(0, CameraType.PERSPECTIVE);
170 previewWorld.SetCameraNearPlane(0, 0.001);
171 previewWorld.SetCameraFarPlane(0, 50);
172 previewWorld.SetCameraVerticalFOV(0, CAMERA_VERTICAL_FOV);
183 if (rscStr ==
string.Empty)
186 Resource rscItem = Resource.Load(rscStr);
187 if (rscItem.IsValid())
204 BaseWorld previewWorld =
m_RTWorld.GetRef();
261 super.OnMapOpen(config);
270 if ( SCR_MapToolInteractionUI.Cast(
m_MapEntity.GetMapUIComponent(SCR_MapToolInteractionUI)) )
272 SCR_MapToolInteractionUI.GetOnDragWidgetInvoker().Insert(
OnDragWidget);
273 SCR_MapToolInteractionUI.GetOnActivateToolInvoker().Insert(
OnActivateTool);
295 super.OnMapClose(config);
302 m_bHookToRoot =
true;
protected void ToggleVisible()
Visibility toggle.
protected SCR_MapEntity m_MapEntity
protected Widget m_RootWidget
protected void SetVisible(bool visible)
protected void OnDragWidget(Widget widget)
protected void OnActivateTool(Widget widget)
SCR_MapToolInteractionUI event.
protected string WIDGET_NAME
ArmaReforgerScripted GetGame()
protected bool SpawnPrefab()
protected void InitPositionVectors()
Initialise RT camera and prefab positon.
IEntity FindRelatedGadget()
protected string GetPrefabResource()
override void OnMapClose(MapConfiguration config)
override void OnMapOpen(MapConfiguration config)
UI Textures DeployMenu Briefing conflict_HintBanner_1_UI desc
protected int m_iSizesCount
SCR_GenericBoxEntityClass GenericEntity
Map tool menu entry data class.
protected vector m_vCameraPos
protected void SetSize(bool nextSize=false)
protected ref SharedItemRef m_RTWorld
protected bool SetupRTWorld()
void SetFrameVisible()
TODO Frame is now set through script here instead of directly in callqueue to avoid leak.
protected bool m_bIsDragged
protected string RT_WIDGET_NAME
protected vector m_vPrefabPos
protected SCR_MapToolEntry m_ToolMenuEntry
protected GenericEntity m_RTEntity
protected RenderTargetWidget m_wRenderTarget
protected void SetWidgetNames()
Set widget names.
protected bool m_bWantedVisible
protected vector m_vCameraAngle
protected string WORLD_NAME
protected string WORLD_RESOURCE
class ToolSize Attribute("", UIWidgets.Object, desc:"Array of x*y sizes in unscaled pix")] protected ref array< ref ToolSize > m_aSizesArray
Base map UI component for map tools which are using RenderTargetWidget to display.
protected bool m_bIsVisible
protected EGadgetType m_eGadgetType
protected Widget m_wFrame
protected int m_iCurrentSizeIndex
SCR_AIGoalReaction_Follow BaseContainerProps
Handles insects that are supposed to be spawned around selected prefabs defined in prefab names array...