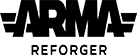 |
Arma Reforger Explorer
1.1.0.42
Arma Reforger Code Explorer by Zeroy - Thanks to MisterOutofTime
|
Go to the documentation of this file.
37 static bool s_bIsInit;
62 protected ref ScriptInvokerBase<OnEntriesChanged>
m_OnEntriesChanged =
new ScriptInvokerBase<OnEntriesChanged>();
64 protected ref ScriptInvokerBase<OnVONActiveToggled>
m_OnVONActiveToggled =
new ScriptInvokerBase<OnVONActiveToggled>();
145 for (
int i = 0; i < count; i++)
200 entry.SetActive(
true);
213 if (radioEntry && !radioEntry.IsLongRange())
261 if (vonRadial && vonRadial.IsOpened())
282 if (reason == EActionTrigger.DOWN)
292 if (reason == EActionTrigger.DOWN)
302 if (reason == EActionTrigger.DOWN)
317 if (reason == EActionTrigger.PRESSED)
327 if (reason == EActionTrigger.PRESSED)
337 if (reason == EActionTrigger.PRESSED)
516 if (!
GetGame().GetVONCanTransmitCrossFaction())
577 if (entry.GetVONMethod() ==
ECommMethod.SQUAD_RADIO)
611 SetVONComponent(SCR_VoNComponent.Cast(to.FindComponent(SCR_VoNComponent)));
617 ChimeraCharacter character = ChimeraCharacter.Cast(from);
626 bool unconsciousVONEnabled;
631 SCR_GameModeHealthSettings healthSettingsComp = SCR_GameModeHealthSettings.Cast(baseGameMode.GetGameModeHealthSettings());
632 if (healthSettingsComp)
633 unconsciousVONEnabled = healthSettingsComp.IsUnconsciousVONPermitted();
635 if (unconsciousVONEnabled)
640 ChimeraCharacter character = ChimeraCharacter.Cast(to);
718 SetVONComponent(SCR_VoNComponent.Cast(controlledEnt.FindComponent(SCR_VoNComponent)));
756 protected void Init(IEntity owner)
758 if (s_bIsInit || System.IsConsoleApp())
784 playerController.m_OnDestroyed.Insert(
OnDestroyed);
831 IEntity ent = PlayerController.Cast(
GetOwner()).GetControlledEntity();
834 ChimeraCharacter character = ChimeraCharacter.Cast(ent);
852 DbgUI.Begin(
"VON debug");
853 string dbg =
"VONcomp: %1 | entries: %2";
855 string dbg2 =
"Gpad Direct mode: %1 | Gpad LRR mode: %2";
857 string dbg3 =
"Activ: %1";
859 string dbg4 =
"LRRad: %1";
861 string dbg5 =
"Saved: %1";
864 string line =
"freq: %2 | active: %3 | class: %4 | type: %1 ";
868 DbgUI.Text(
string.Format( line,
SCR_Enum.GetEnumName(EGadgetType, radio.GetGadget().GetType()), radio.GetEntryFrequency(), radio.IsActive(), radio.ToString()));
898 World world =
GetOwner().GetWorld();
903 updateSystem.Register(
this);
909 World world =
GetOwner().GetWorld();
914 updateSystem.Unregister(
this);
952 super.OnDelete(owner);
bool SetVONDisabled(bool state)
ScriptInvokerBase< OnVONActiveToggled > GetOnVONActiveToggledInvoker()
protected void ActivateVON(EVONTransmitType transmitType)
protected SCR_VONEntry m_SavedEntry
void SetDisplay(SCR_VonDisplay display)
protected void SetGamepadLRRMode(bool state, bool isSwitch=false)
Sets long rang radio mode for gamepad input scheme.
protected bool m_bIsUsingGamepad
SCR_VONEntry GetActiveEntry()
protected void VONChannel(bool activate)
int GetVONEntryCount()
Get number of entries.
protected EVONTransmitType m_eVONType
protected InputManager m_InputManager
protected void OnPlayerDeleted(int playerId, IEntity player)
int GetVONEntries(inout array< ref SCR_VONEntry > entries)
SCR_SiteSlotEntityClass ScriptComponent
protected void OnControlledEntityChanged(IEntity from, IEntity to)
Runs every time the controlled entity has been changed.
protected void OnInputDeviceIsGamepad(bool isGamepad)
Game Event.
protected bool m_bIsActiveModeLong
SCR_VonDisplay GetDisplay()
bool AssignVONComponent()
Assign VON comp by fetching it from controlled entity.
ArmaReforgerScripted GetGame()
protected void OnVONToggle(float value, EActionTrigger reason)
VON toggle val 0 = off / 1 = direct / 2 = channel.
protected void DeactivateVON(EVONTransmitType transmitType=EVONTransmitType.NONE)
Current VON deactivation.
protected bool m_bIsPauseDisabled
void SetVONComponent(SCR_VoNComponent VONComp)
protected void Init(IEntity owner)
Initialize component, done once per controller.
EVONTransmitType
Type of a players current VON transmission.
override void OnDelete(IEntity owner)
protected void Cleanup()
Cleanup component.
protected void ConnectToHandleUpdateVONControllersSystem()
protected ref SCR_VONMenu m_VONMenu
protected void OnVONLongRange(float value, EActionTrigger reason)
VON channel speech listener callback for long range radios.
protected bool m_bIsToggledChannel
const protected string VON_DIRECT_HOLD
protected void SetActiveTransmit(notnull SCR_VONEntry entry)
Set transmission method depending on entry type when starting VON transmit.
VONEntry class for radio entries.
protected string m_sActiveHoldAction
ScriptInvokerBase< OnEntriesChanged > GetOnEntriesActiveChangedInvoker()
proto external PlayerController GetPlayerController()
protected SCR_VONEntry m_LongRangeEntry
SCR_BaseGameMode GetGameMode()
protected void OnVONSwitch(float value, EActionTrigger reason)
VON switch between direct/channel mode.
protected ref array< ref SCR_VONEntry > m_aEntries
protected void UpdateSystemState()
protected ref ScriptInvokerBase< OnEntriesChanged > m_OnEntriesActiveChanged
protected SCR_VonDisplay m_VONDisplay
const protected float TOGGLE_OFF_DELAY
protected void OnVONGamepad(float value, EActionTrigger reason)
VON channel speech listener callback.
protected SCR_VONEntry GetEntryByTransmitType(EVONTransmitType type)
protected void VONLongRange(bool activate)
bool IsVONDisabled()
Is VON disabled.
const protected string VON_LONG_RANGE_HOLD
override protected void EOnDeactivate(IEntity owner)
protected bool m_bIsUnconscious
protected void UpdateDebug()
Debug.
protected bool m_bIsToggledDirect
bool IsUsingLRR()
Using long range radio.
typedef Attribute
Post-process effect of scripted camera.
protected void OnVONLongRangeGamepadToggle(float value, EActionTrigger reason)
protected bool m_bIsActive
protected void OnVONToggleGamepad(float value, EActionTrigger reason)
VON toggle controller.
protected ref ScriptInvokerBase< OnVONActiveToggled > m_OnVONActiveToggled
override protected void OnPostInit(IEntity owner)
Editable Mine.
protected void OnVONChannel(float value, EActionTrigger reason)
VON channel speech listener callback.
protected ref SCR_VONEntry m_DirectSpeechEntry
protected void Deactivate()
IEntity GetOwner()
Owner entity of the fuel tank.
protected bool m_bIsActiveModeDirect
bool IsLRRAvailable()
Long range raido entry is available.
protected float m_fToggleOffDelay
void RemoveEntry(SCR_VONEntry entry)
protected void OnDestroyed(Instigator killer, IEntity killerEntity)
protected SCR_VoNComponent m_VONComp
protected bool m_bIsDisabled
protected SCR_VONEntry m_ActiveEntry
protected void OnVONDirect(float value, EActionTrigger reason)
VON direct speech listener callback.
void SetEntryActive(SCR_VONEntry entry, bool setFromMenu=false)
void Update(float timeSlice)
protected void OnPauseMenuClosed()
PauseMenuUI Event.
protected void TimeoutVON()
VON hold timeout, stops active von transmission in case of specific condition which would prevent for...
SCR_VoNComponent GetVONComponent()
Get active VON component for transmit.
void SCR_FactionManager(IEntitySource src, IEntity parent)
protected void OnPauseMenuOpened()
PauseMenuUI Event.
protected void DisconnectFromHandleUpdateVONControllersSystem()
protected void VONDirect(bool activate)
protected string m_sLocalEncryptionKey
protected ref ScriptInvokerBase< OnEntriesChanged > m_OnEntriesChanged
void OnLifeStateChanged(ECharacterLifeState previousLifeState, ECharacterLifeState newLifeState)
Will be called when the life state of the character changes.
Voice over network entry data class, used for management of communication methods.
const protected string VON_CHANNEL_HOLD
void AddEntry(SCR_VONEntry entry)
protected void InitEncryptionKey()
proto external int GetPlayerId()
SCR_VONControllerClass VON_MENU_OPENING_CONTEXT
Scripted VON input and control, attached to SCR_PlayerController.
ScriptInvokerBase< OnEntriesChanged > GetOnEntriesChangedInvoker()