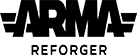 |
Arma Reforger Explorer
1.1.0.42
Arma Reforger Code Explorer by Zeroy - Thanks to MisterOutofTime
|
Go to the documentation of this file.
25 protected ref array<ref SCR_BaseNetworkedStat> m_aStats;
28 [
Attribute(
"3", uiwidget: UIWidgets.EditBox,
desc:
"How many seconds should we wait before checking and updating the stats again?")]
38 if (stat.GetStatType() == statType)
73 [
RplRpc(RplChannel.Unreliable, RplRcver.Broadcast)]
80 networkedStat.OnAuthorityStatValueSet(value);
101 m_RplComponent = RplComponent.Cast(owner.FindComponent(RplComponent));
107 networkedStat.SetOwner(
this);
114 SetEventMask(owner, EntityEvent.INIT);
SCR_FragmentEntityClass ComponentEditorProps
SCR_NetworkedStatsComponentClass GetNetworkedStatPrefabData()
ScriptInvokerBase< SCR_OnStatChangedMethod > SCR_OnStatChangedInvoker
void SetStatValueOfAuthority(SCR_ENetworkedStatType statType, int value)
SCR_SiteSlotEntityClass ScriptComponent
func SCR_OnStatChangedMethod
ArmaReforgerScripted GetGame()
override void OnDelete(IEntity owner)
UI Textures DeployMenu Briefing conflict_HintBanner_1_UI desc
SCR_AchievementsHandlerClass ScriptComponentClass RplRpc(RplChannel.Reliable, RplRcver.Owner)] void UnlockOnClient(AchievementId achievement)
RplComponent GetRplComponent()
Returns the replication component associated to this entity.
SCR_NetworkedStatsComponentClass ScriptComponentClass Attribute()] protected ref array< ref SCR_BaseNetworkedStat > m_aStats
Post-process effect of scripted camera.
override void OnPostInit(IEntity owner)
Editable Mine.
protected ref SCR_OnStatChangedInvoker m_OnStatsChanged
IEntity GetOwner()
Owner entity of the fuel tank.
void SetAuthorityStatValue(SCR_ENetworkedStatType statType, int valueInt)
override void EOnInit(IEntity owner)
Initialise this component with data from FactionsManager.
protected RplComponent m_RplComponent
SCR_BaseNetworkedStat GetNetworkedStat(SCR_ENetworkedStatType statType)
protected int m_iUpdateTimer