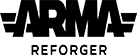 |
Arma Reforger Explorer
1.1.0.42
Arma Reforger Code Explorer by Zeroy - Thanks to MisterOutofTime
|
Go to the documentation of this file. 1 [
ComponentEditorProps(
category:
"GameScripted/Character", description:
"Scripted character command handler", icon: HYBRID_COMPONENT_ICON)]
6 class SCR_CharacterCommandHandlerComponent : CharacterCommandHandlerComponent
11 if (pInputCtx.GetMeleeAttack())
14 pInputCtx.SetMeleeAttack(
false);
41 super.OnCommandActivate(pCmdId);
63 if (!currentCmdScripted)
84 [
RplRpc(RplChannel.Reliable, RplRcver.Server)]
100 override protected void OnInit(IEntity owner)
112 Print(
"Failed to bind scripted static table (see class SCR_ScriptedCommandsStaticTable). This can be caused by missing animation commands, tags, or events in the animation graph.");
125 protected ref CharacterCommandMoveSettings
m_MoveSettings =
new CharacterCommandMoveSettings();
SCR_FragmentEntityClass ComponentEditorProps
protected ChimeraCharacter m_OwnerEntity
protected ref CharacterCommandMoveSettings m_MoveSettings
protected SCR_CharacterCommandLoiter GetLoiterCommand()
proto external CharacterCommandMelee GetCommandModifier_Melee()
void Rpc_StopLoiter_S(bool terminateFast)
ScriptInvokerBase< ScriptInvokerIntMethod > ScriptInvokerInt
void StartCommandLoitering()
override void OnCommandActivate(int pCmdId)
ScriptInvokerInt GetOnCommandActivate()
protected ref CharacterCommandClimbSettings m_ClimbSettings
SCR_AchievementsHandlerClass ScriptComponentClass RplRpc(RplChannel.Reliable, RplRcver.Owner)] void UnlockOnClient(AchievementId achievement)
proto external CharacterInputContext GetInputContext()
protected BaseWeaponManagerComponent m_WeaponManager
protected ref SCR_ScriptedCommandsStaticTable m_ScrStaticTable
protected CharacterControllerComponent m_CharacterControllerComp
void StopLoitering(bool terminateFast)
protected CharacterAnimationComponent m_CharacterAnimComp
protected SCR_MeleeComponent m_MeleeComponent
class SCR_ScriptedCommandsStaticTable SCR_CharacterCommandLoiter
CharacterCommandHandlerComponentClass BaseCommandHandlerComponentClass GetControllerComponent()
Returns the current character controller component.
protected ref CharacterMovementState m_MovementState
override protected void OnInit(IEntity owner)
protected ref ScriptInvokerInt m_OnCommandActivate
protected ref SCR_CharacterCommandLoiter m_CmdLoiter
SCR_CharacterCommandHandlerComponentClass CharacterCommandHandlerComponentClass HandleMelee(CharacterInputContext pInputCtx, float pDt, int pCurrentCommandID)