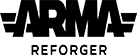 |
Arma Reforger Explorer
1.1.0.42
Arma Reforger Code Explorer by Zeroy - Thanks to MisterOutofTime
|
Go to the documentation of this file.
16 class CharacterControllerComponent: PrimaryControllerComponent
31 proto external
void SetMovement(
float movementSpeed, vector movementDirModel);
32 proto external
void SetHeadingAngle(
float newHeadingAngle,
bool adjustAimingYaw =
false);
40 proto external
void SetThrow(
bool val,
bool cancelThrow);
48 proto external
void SetFreeLook(
bool input,
bool mouse,
bool controller);
88 proto external
void SetInspect(IEntity targetItem);
93 proto external
bool CanInspect(IEntity targetItem);
139 proto external
void SetRoll(
int val);
140 proto external
bool IsRoll();
141 proto external
bool CanRoll(
int val);
145 proto external
void SetJump(
float val);
175 proto external
bool IsDead();
191 proto external
bool SetSafety(
bool safety,
bool automatic);
197 proto external
bool ReloadWeaponWith(IEntity ammunitionEntity,
bool bForceDetach =
false);
207 proto external
void Ragdoll(
bool broadcast =
true);
217 proto external
void TakeGadgetInLeftHand(IEntity gadget,
int gadgetType,
bool autoFocus =
false,
bool skipAnimations =
false);
228 proto external
bool TryUseBandage(IEntity bandage,
int bodyPart,
float duration = 4.0,
bool bandageSelf =
true);
249 proto external
bool TryUseItem(IEntity item,
bool allowMovementDuringAction =
false,
bool keepInHandAfterSuccessfulAction =
false);
281 proto external
bool CanUseLadder(IEntity pLadderOwner,
int ladderComponentIndex = 0,
float maxTestDistance = -1.0,
float maxEntryAngle = -1.0,
bool performTraceCheck =
false);
284 proto external
bool TryUseLadder(IEntity pLadderOwner,
int ladderComponentIndex = 0,
float maxTestDistance = -1.0,
float maxEntryAngle = -1.0);
367 [
Obsolete(
"This method will be removed soon!")]
368 proto external
void GetWeaponModifiers(
float baseScale,
float moveScale, out vector scaleA, out vector scaleB);
384 event protected void OnInit(IEntity owner);
390 event protected void OnDiag(IEntity owner,
float timeslice);
392 event protected void OnPrepareControls(IEntity owner, ActionManager am,
float dt,
bool player);
409 event protected void OnDeath(IEntity instigatorEntity, notnull
Instigator instigator);
418 event protected void OnAnimationEvent(AnimationEventID animEventType, AnimationEventID animUserString,
int intParam,
float timeFromStart,
float timeToEnd);
proto external bool GetFreeLookController()
proto external bool IsFalling()
proto external bool TryUseItem(IEntity item, bool allowMovementDuringAction=false, bool keepInHandAfterSuccessfulAction=false)
proto external bool IsWeaponRaised()
proto external float GetStanceChangeDelayTime()
proto external void GetWeaponModifiers(float baseScale, float moveScale, out vector scaleA, out vector scaleB)
proto external bool GetMouseControlAircraft()
proto external IEntity GetCurrentItemInHands()
proto external bool CanRoll(int val)
proto external bool CanReviveCharacter()
event protected bool OnPerformAction()
Override to handle what happens after pressing F button, return false to use default cpp behavior.
event protected void OnPrepareControls(IEntity owner, ActionManager am, float dt, bool player)
proto external bool IsPlayerControlled()
proto external bool TryUseLadder(IEntity pLadderOwner, int ladderComponentIndex=0, float maxTestDistance=-1.0, float maxEntryAngle=-1.0)
proto external float GetJumpSpeed()
event protected void OnConsciousnessChanged(bool conscious)
Will be called when the consciousness of the character changes.
event bool ShouldAligningAdjustAimingAngles()
proto external EntitySlotInfo GetRightHandPointInfo()
proto external IEntity GetInspectEntity()
proto external bool IsReloading()
proto external void SetWeaponNoFireTime(float t)
proto external float GetMovementSpeed()
CharacterControllerComponentClass PrimaryControllerComponentClass GetAimingComponent()
proto external int GetCurrentMovementPhase()
proto external bool IsUnconscious()
proto external vector GetMovementInput()
proto external CameraHandlerComponent GetCameraHandlerComponent()
proto external bool CanFire()
proto external void SetStickyGadget(bool enable)
event bool SCR_GetDisableViewControls()
proto external bool CanInspect(IEntity targetItem)
proto external void SetUnconscious(bool enabled)
proto external float GetDynamicSpeed()
proto external IEntity GetRightHandItem()
Returns generic item attached to right hand. Returns null if there's none (or if active item is weapo...
proto external void SetDisableViewControls(bool other)
proto external bool TryUseBandage(IEntity bandage, int bodyPart, float duration=4.0, bool bandageSelf=true)
proto external void EnableReviveCharacter(bool enabled)
proto external bool CanChangeStance(int stance)
CharacterStanceChange STANCECHANGE_NONE = 0, STANCECHANGE_TOERECTED = 1, STANCECHANGE_TOCROUCH = 2,...
proto external AIControlComponent GetAIControlComponent()
event protected void OnReloaded(IEntity owner, BaseWeaponComponent weapon)
proto external void SetJump(float val)
event void SCR_OnDisabledJumpAction()
proto external bool GetInspect()
proto external ECharacterStance GetStance()
Returns the current stance of the character.
proto external bool IsUsingItem()
proto external bool IsPlayingGesture()
proto external void SetMeleeAttack(bool val)
proto external void SetWeaponADSInput(bool val)
proto external void SetSightsRange(int index)
event bool CanJumpOutVehicleScript()
Override to handle whether character can eject from vehicle via JumpOut input action.
event protected void OnGadgetStateChanged(IEntity gadget, bool isInHand, bool isOnGround)
Will be called when gadget taken/removed from hand.
proto external bool SetGadgetRaisedModeWanted(bool newRaised)
Returns true if focus will be changed to requested.
proto external InventoryStorageManagerComponent GetInventoryStorageManager()
proto external void StopDeployment()
proto external void SetInspectState(int state)
event bool GetCanEquipGadget(IEntity gadget)
proto external bool IsFocusMode()
proto external void SetShouldApplyDynamicSpeedOverride(bool shouldApply)
event bool SCR_GetDisableMovementControls()
proto external bool IsCharacterStationary()
proto external vector GetVelocity()
proto external bool IsChangingStance()
proto external bool IsInThirdPersonView()
proto external void TakeGadgetInLeftHand(IEntity gadget, int gadgetType, bool autoFocus=false, bool skipAnimations=false)
proto external bool IsMeleeAttack()
proto external void RequestActionByID(int actionID, float value)
proto external void SetFreeLook(bool input, bool mouse, bool controller)
event bool ShouldGadgetBeDropped(IEntity gadget)
proto external vector GetMovementDirWorld()
proto external bool SetFireMode(int index)
proto external float GetADSTime()
proto external void SetDisableMovementControls(bool other)
proto external ECharacterLifeState GetLifeState()
proto external CharacterInputContext GetInputContext()
event protected void OnItemUseEnded(ItemUseParameters itemUseParams, bool successful)
Will be called when item use action is complete.
proto external bool SetSafety(bool safety, bool automatic)
proto external void DropWeapon(WeaponSlotComponent weaponSlot)
Makes character drop weapon from the weapon slot given by parameter.
Adds ability to attach an object to a slot.
proto external bool CanSetDynamicStance(float value)
proto external void SetStickyADS(bool enable)
proto external void StopCharacterGesture()
proto external bool IsGadgetInHands()
Returns true if there is a gadget in hands.
proto external bool IsGadgetRaisedModeWanted()
Returns true if character will be (or is) using gadget in raised mode.
event protected void OnMeleeDamage(bool started)
Handling of melee events. Sends true if melee started, false, when melee ends.
proto external void SetStanceChange(int stance)
CharacterStanceChange STANCECHANGE_NONE = 0, STANCECHANGE_TOERECTED = 1, STANCECHANGE_TOCROUCH = 2,...
proto external void SetDynamicStance(float value)
proto external bool SetMuzzle(int index)
proto external bool TryUseItemOverrideParams(notnull ItemUseParameters params)
proto external float GetLastStanceChangeDelay()
proto external bool IsChangingItem()
proto external void ForceDeath()
Kills the character. Skips invincibility checks.
proto external float GetDynamicStance()
proto external bool IsTrackIREnabled()
proto external SCR_ChimeraCharacter GetCharacter()
Returns the current controlled character.
proto external bool TryStartCharacterGesture(int gesture, int durationMS=0)
Starts character gesture with specified duration in milliseconds (if duration <= 0,...
proto external bool CanUseItem()
Returns true if the character can use an item.
proto external BaseWeaponManagerComponent GetWeaponManagerComponent()
proto external void SetBanking(float val)
proto external void ResetPersistentStates(bool resetADSState=true, bool resetGadgetState=true)
proto external bool ReloadWeaponWith(IEntity ammunitionEntity, bool bForceDetach=false)
event void UpdateDrowning(float timeSlice, vector waterLevel)
event protected void OnControlledByPlayer(IEntity owner, bool controlled)
Called when a player has been assigned to this controller.
event float GetInspectTargetLookAt(out vector targetAngles)
proto external bool CanUseLadder(IEntity pLadderOwner, int ladderComponentIndex=0, float maxTestDistance=-1.0, float maxEntryAngle=-1.0, bool performTraceCheck=false)
proto external void SetForcedFreeLook(bool enabled)
Force character to stay in freelook.
proto external bool IsChangingFireMode()
proto external bool CanEnterUnconsciousness()
proto external bool GetCanThrow()
proto external bool IsFreeLookEnforced()
Returns true if freelook is enforced by game logic.
proto external IEntity GetAttachedGadgetAtLeftHandSlot()
event protected void OnDiag(IEntity owner, float timeslice)
proto external EWeaponObstructedState GetWeaponObstructedState()
proto external bool IsSwimming()
proto external void SetMouseControlAircraft(bool enable)
proto external bool ShouldHoldInputForRoll()
proto external void DropItemFromLeftHand()
Makes character drop item from left hand.
proto external bool GetIsSprintingToggle()
proto external bool GetMaxZoomInADS()
proto external CharacterAnimationComponent GetAnimationComponent()
proto external void RemoveGadgetFromHand(bool skipAnimations=false)
Remove held gadget.
event void OnInspectionModeChanged(bool newState)
proto external void SetDisableWeaponControls(bool other)
proto external bool ReloadWeapon()
Request weapon reload. If true, request was sucessful.
proto external void SetInThirdPersonView(bool state)
proto external vector GetMovementVelocity()
proto external void SetAimingSensitivity(float mouse, float gamepad, float ads)
proto external bool CanEquipGadget(IEntity gadget)
proto external void SetRoll(int val)
2 - right, 1 - left
proto external float GetWantedLeaning()
proto external bool IsLeaning()
proto external bool GetFreeLookMouse()
proto external bool IsPlayingItemGesture()
Returns true if the character is playing a gesture.
proto external float GetStamina()
event protected void OnDeath(IEntity instigatorEntity, notnull Instigator instigator)
Handling of death. If instigatorEntity is null, you can use instigator.GetInstigatorEntity() if appro...
proto external void ForceStanceUp(int stance)
proto external void RecoverHiddenGadget(bool respectSettings, bool skipAnims)
Put held gadget on hold.
proto external float GetObstructionAlpha()
proto external vector GetCameraWeaponOffset()
proto external void ForceStance(int stance)
proto external bool GetFreeLookInput()
event bool CanGetOutVehicleScript()
Override to handle whether character can get out of vehicle via GetOut input action.
proto external float GetLeaning()
Either character wants to lean.
proto external void SetDynamicSpeed(float value)
proto external void SetThrow(bool val, bool cancelThrow)
Set wanted input action values.
SCR_DestructionSynchronizationComponentClass ScriptComponentClass int index
proto external bool GetIsWeaponDeployed()
proto external bool IsFreeLookEnabled()
proto external bool CanEngageChangeItem()
proto external void SetPartialLower(bool state)
Sets desired partial lower state, if allowed.
proto external bool IsWeaponADS()
proto external void SetWeaponADS(bool val)
Set the current weapon ADS state.
proto external void SetWeaponRaised(bool val)
Set the current weapon-raised state.
proto external bool GetPositionInView(vector pos, float angMax)
proto external bool GetIsWeaponDeployedBipod()
proto external bool GetWeaponADSInput()
proto external bool GetInspectCurrentWeapon()
proto external void GetAimingSensitivity(out float mouse, out float gamepad, out float ads)
proto external bool GetDisableWeaponControls()
proto external bool GetDisableMovementControls()
proto external bool GetDisableViewControls()
proto external EntitySlotInfo GetLeftHandPointInfo()
Configs ServerBrowser KickDialogs params
proto external bool SelectWeapon(BaseWeaponComponent newWeapon)
Set weapon on character with switching animations. If true, the request was successful.
proto external int GetInspectState()
proto external bool TryPlayItemGesture(EItemGesture gesture, BaseUserAction callbackAction=null, string confirmEvent="")
proto external VoNComponent GetVONComponent()
Get active VON component for transmit.
proto external bool CanPartialLower()
Returns true if the character can partially lower (weapon).
protected override event void OnAnimationEvent(AnimationEventID animEventType, AnimationEventID animUserString, int intParam, float timeFromStart, float timeToEnd)
event protected void OnWeaponDropped(IEntity pWeaponEntity, WeaponSlotComponent pWeaponSlot)
Runs after a weapon is dropped from hands. Returns dropped weapon entity and slot that the weapon was...
proto external void SetMovementDirWorld(vector movementDirWorld)
Update simulation state with difference of world position.
proto external bool CanDeployWeapon()
proto external CharacterHeadAimingComponent GetHeadAimingComponent()
proto external void SetWantedLeaning(float val)
proto external void SetMaxZoomInADS(bool enable)
proto external void Ragdoll(bool broadcast=true)
Dying.
proto external CharacterStaminaComponent GetStaminaComponent()
proto external bool TryEquipRightHandItem(IEntity item, EEquipItemType type, bool swap=false, BaseUserAction callbackAction=null)
proto external void SetMovement(float movementSpeed, vector movementDirModel)
Update animation about state of movement, define speed and direction in local space of character.
override protected void OnInit(IEntity owner)
proto external bool IsSprinting()
proto external void SetFireWeaponWanted(bool val)
Set wanted input action values.
proto external bool IsClimbing()
proto external bool IsDead()
event protected void OnLifeStateChanged(ECharacterLifeState previousLifeState, ECharacterLifeState newLifeState)
Will be called when the life state of the character changes.
proto external bool IsFreeLookForced()
proto external bool CanPlayItemGesture()
Returns true if the character can play a gesture.
proto external bool TryRecoverLastRightHandItem(bool swap=false, BaseUserAction callbackAction=null)
proto external void SetInspect(IEntity targetItem)
proto external bool IsAdjustingLeaning()
proto external bool GetMeleeAttackInput()
RespawnSystemComponentClass GameComponentClass Obsolete()] proto external GenericEntity DoSpawn(string prefab
RespawnSystemComponent should be attached to a gamemode to handle player spawning and respawning.
event protected void OnApplyControls(IEntity owner, float timeSlice)
proto external float GetCurrentLeanAmount()
Returns current amount of leaning applied.
proto external void EnableHoldInputForRoll(bool enable)
event protected void OnGadgetFocusStateChanged(IEntity gadget, bool isFocused)
Will be called when gadget fully transitioned to or canceled focus mode.
proto external void SetHeadingAngle(float newHeadingAngle, bool adjustAimingYaw=false)
proto external bool IsRoll()
event protected void OnItemDroppedFromLeftHand(IEntity pItemEntity)
Runs after the left hand item is dropped. Returns dropped item entity.
proto external bool GetCanFireWeapon()
proto external bool IsPartiallyLowered()
Returns true if the character is partially lowered.
event protected void OnItemUseBegan(ItemUseParameters itemUseParams)
Will be called when item use action is started.
event bool GetCanMeleeAttack()